Here is the shader pair:
varying vec4 v;
void main(){ v = gl_Position = ftransform(); }
The vertex shader assumes something is drawn on the screen (a quad say) and covers the screen as a surface for the distortion shader. This means v will hold co-ordinates -1.0..1.0
uniform sampler2D s;
varying vec4 v;
void main(){
vec2 x = v.xy/2.0 + 0.5;
gl_FragColor = texture2D( s, x + 0.1*tan(x*5.0) );
}
Firstly v is mapped to 0.0..1.0 range of normal texture co-ordinates. The glass tile effect is then simply that tan distortion! Here is an image of what it can produce - ignore the background, thats just something to be distorted.
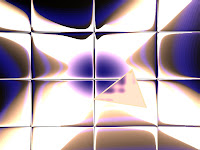
If you want to "waste" bytes, its a good idea to do something like:
gl_FragColor = texture2D( s, x+min( 0.1*tan(x*5.0), 0.2 ) );
This helps to prevent "sparklies" in the distortion by keeping that tan under control.